Understanding Microservices in .NET Core
Microservices have revolutionized the way applications are designed and developed. With the advent of cloud computing and the need for scalable, maintainable, and resilient applications, microservices architecture has become a go-to solution for many enterprises. In this blog, we will delve into microservices in .NET Core, exploring their benefits, architecture, implementation, and best practices.
What are Microservices?
Microservices represent an architectural style where an application consists of a set of small, self-contained services that communicate through APIs. Each service is built for a specific task and can be developed, deployed, and scaled on its own.
Why Choose Microservices?
Scalability: Each service can be scaled independently to meet varying demands.
Technology Flexibility: Services can utilize diverse programming languages or technologies as needed.
Enhanced Resilience: Issues in one service won’t disrupt the entire system.
Accelerated Development: Multiple teams can work on separate services at the same time.
Better Maintainability: Smaller, independent codebases are easier to work with and update.
Microservices Architecture
A microservices architecture comprises various components in addition to the microservices themselves:
- Management: Oversees and manages the nodes for the services.
- Identity Provider: Handles identity information and provides authentication services across the distributed network.
- Service Discovery: Tracks the services, along with their addresses and endpoints.
- API Gateway: Acts as the client’s primary entry point, serving as a single contact point that retrieves responses from underlying microservices. It can also aggregate responses from multiple microservices.
- CDN (Content Delivery Network): Delivers static resources, such as pages and web content, across a distributed network to enhance performance and reliability.
- Static Content: Refers to unchanging resources like web pages and other content.
Each microservice is deployed independently and maintains its own database, resulting in an architecture that might resemble the illustration shown below.
Monolithic vs Microservices Architecture
Monolithic applications function as a single, comprehensive package that encapsulates all the necessary components and services within one unified system.
The following is a diagrammatic representation of monolithic architecture, showcasing its structure as a fully packaged entity or as a service-based approach.
Microservices is an architectural approach that involves creating small, independent services, each operating in its own environment and communicating through messaging. Each service manages its own database directly.
Below is a diagrammatic representation of a microservices architecture.
In a monolithic architecture, a single database is shared across all functionalities, even when a service-oriented approach is used. In contrast, microservices architecture ensures that each service has its own dedicated database.
Microservice using ASP.NET Core
This section provides a step-by-step guide, with illustrations, to creating a Product microservice using ASP.NET Core. The microservice will be developed using ASP.NET Core 2.1 and Visual Studio 2017, which includes built-in support for ASP.NET Core. The service will feature its own DbContext and database with an isolated repository, enabling independent deployment.
Creating an ASP.NET Core Application Solution
- Open Visual Studio and create a new project.
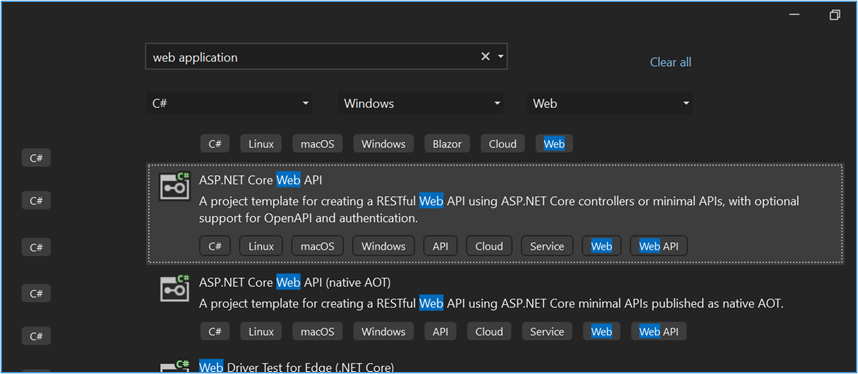
2. Select ASP.NET Core Web API as the project type.
3. Provide a meaningful name for the project.
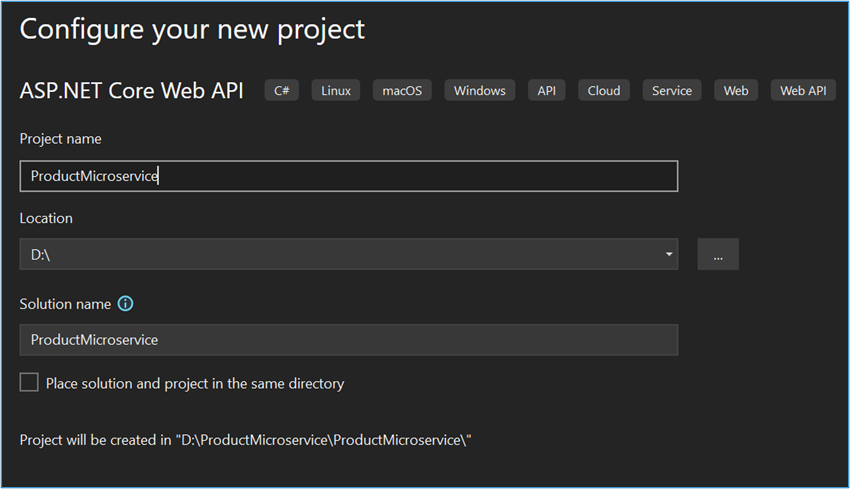
4. Once created, the solution structure will appear as shown below.
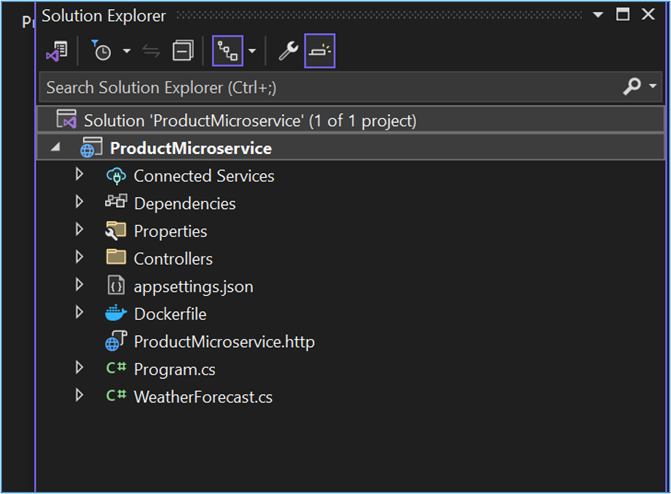
Adding Models
- Add a new folder named “Model” to the project.
- Inside the Models folder, create a Product class.
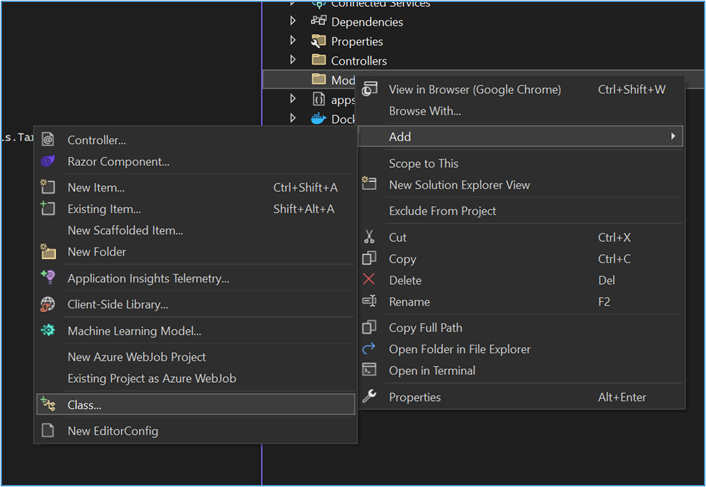
3. Define properties such as Id, Name, Description, and Price in the Product class.
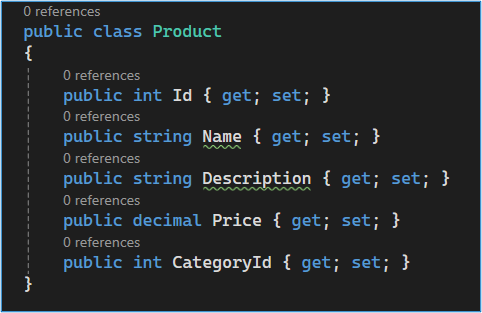
4. To categorize products, create a Category model and add a CategoryId property to the Product model.
5. Similarly, add a Category class in the Models folder.
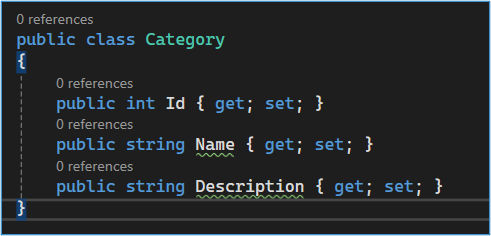
Enabling EF Core
A .NET Core API project comes with built-in support for Entity Framework Core (EF Core), and all necessary dependencies are downloaded automatically during project creation and compilation. These dependencies can be found under the SDK section of the project.
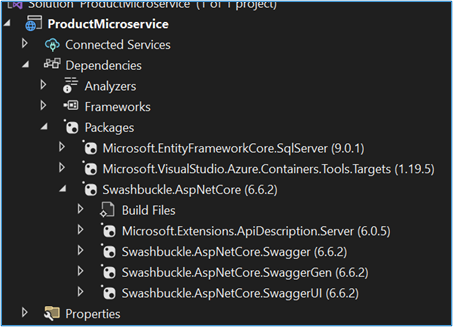
Among the downloaded packages, is Microsoft.EntityFrameworkCore.SqlServer should be present. If it is missing, it can be manually added to the project via NuGet Package Manager.
Adding EF Core DbContext
To enable interaction between the models and the database, a database context is required.
- Create a new folder named DBContexts in the project.
- Add a new class named ProductContext inside the DBContexts
- Inside ProductContext, define DbSet properties for Products and Categories.
- Implement the OnModelCreating method to seed initial master data. Add some sample categories to be inserted into the Category table when the database is created.
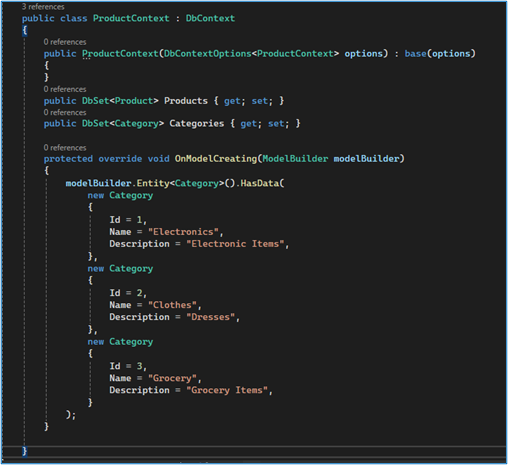
5. Open the appsettings.json file and add a connection string for the database.
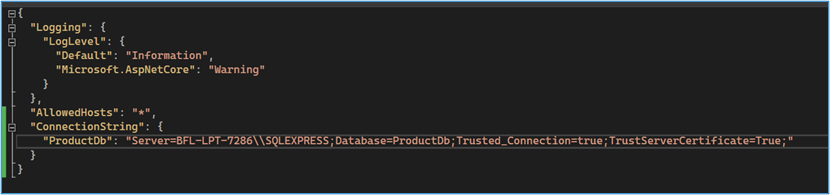
6. Open cs and configure Entity Framework Core to use SQL Server by adding the following code inside the ConfigureServices method:
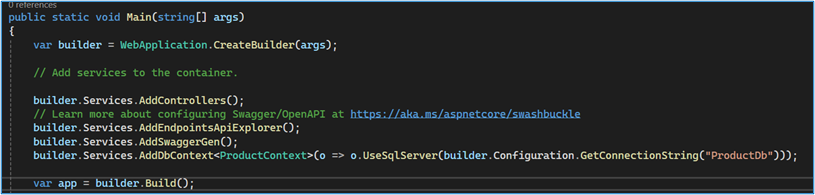
Adding Repository
A repository acts as a micro-component within a microservice, encapsulating the data access layer to improve data persistence and testability.
- Create a new folder named Repository in the project.
- Inside the Repository folder, add an interface named IProductRepository.
- Define methods in IProductRepository to perform CRUD operations for the Product
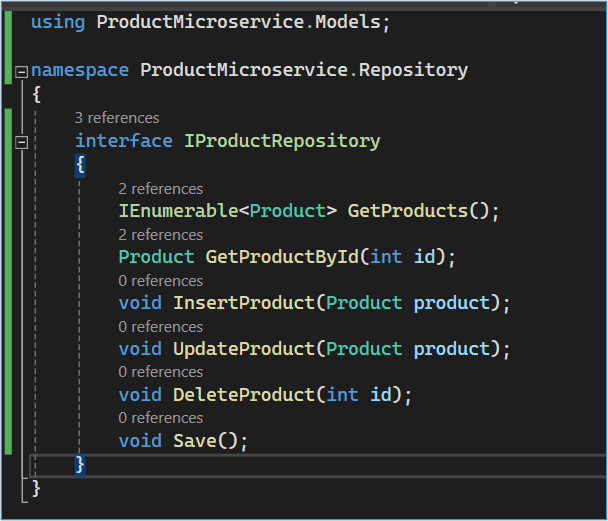
4. Add a new class named ProductRepository in the Repository folder that implements IProductRepository.
5. Implement the CRUD methods in ProductRepository by accessing the database context methods.
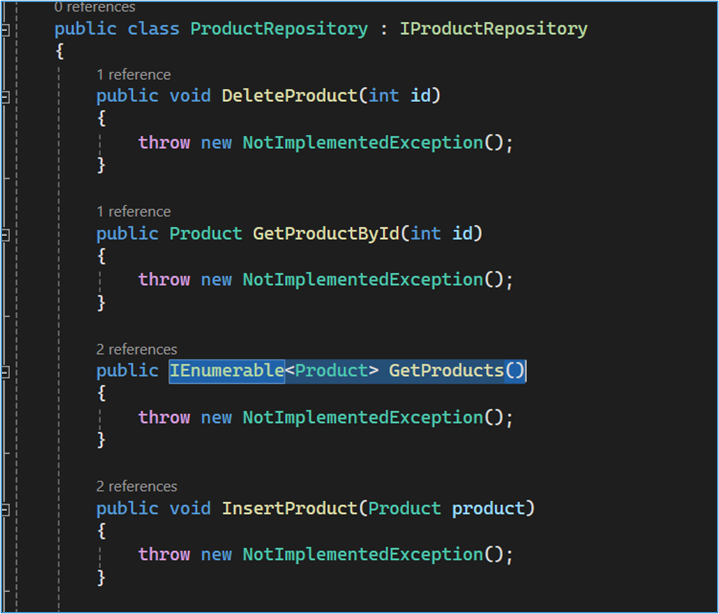
6. Open cs and add the following code inside the ConfigureServices method to enable dependency injection:
Add a Controller:
To create an endpoint for the microservice, a controller is required to expose HTTP methods as service endpoints for the client.
- Right-click on the Controllers folder and select Add → Controller.
- Choose "API Controller with read/write actions" as the template.
- Name the controller ProductController.
- This will generate a ProductController class inside the Controllers folder, containing default read/write actions. These methods will later be replaced with product-specific read/write actions. The HTTP methods in the controller will serve as the service’s endpoints.
- Next, implement the methods by calling the repository methods, as shown below. This basic implementation helps illustrate the concept. Additionally, methods can be attribute-routed and decorated with annotations as needed.
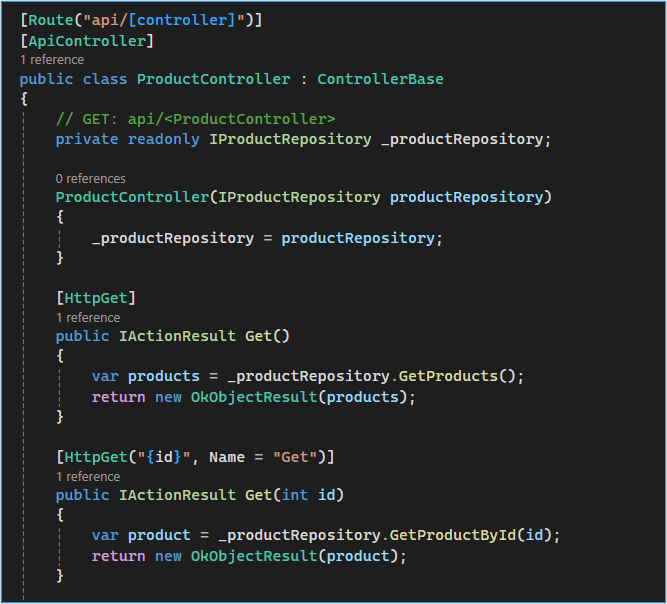
Entity Framework Core Migrations
Migrations allow us to define and apply changes to the database structure as it evolves from one version to another.
Steps to Enable and Apply Migrations:
1. Open Package Manager Console.
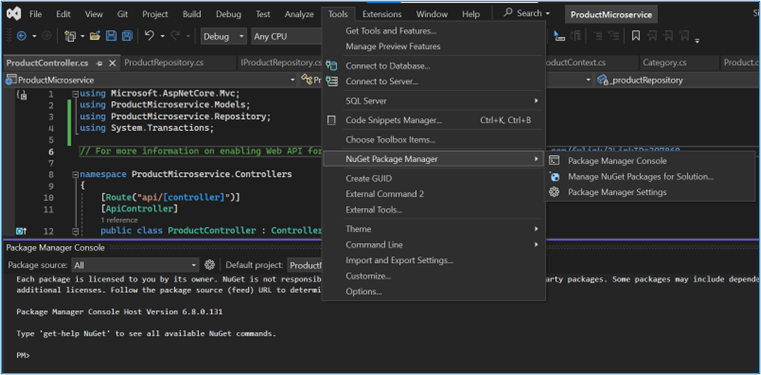
2. To enable migrations, run the following command:
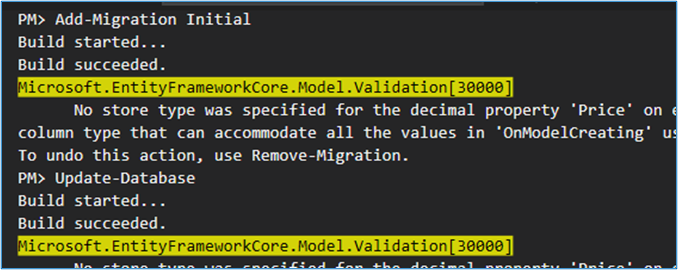
3. Once executed, a new Migrations folder will be created in the solution. It contains
A migration file defining the changes.
A snapshot of the current context model.
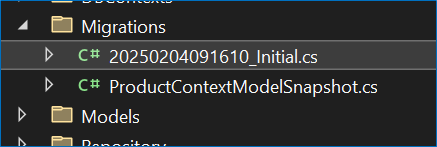
4. To apply the migration to the database, run

5. Check the SQL Server Management Studio to verify if the database got created.
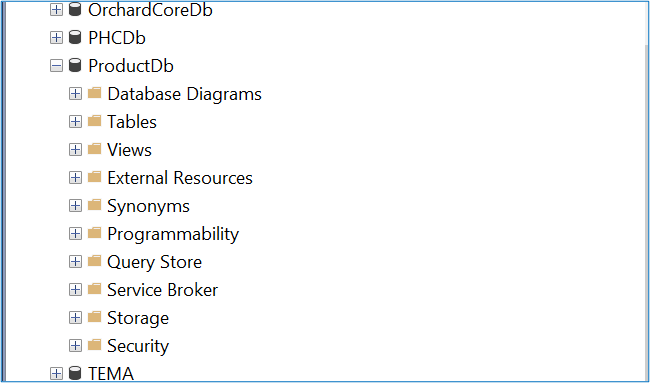
This command ensures the migrations are applied to the current database.
Finally, open SQL Server Management Studio (SSMS) to verify that the database has been created and updated accordingly.
Conclusion
A microservice is a service built around a specific business capability and can be independently deployed, a concept known as bounded context. This article on microservices explores their definition and advantages over monolithic architecture. It provides a detailed guide on developing a microservice using ASP.NET Core and running it via IIS and Docker containers. Additionally, the article explains how a service can have multiple images and run on multiple containers simultaneously.