Implementation of Local Storage and Session Storage in JavaScript
Web Storage Evolution:
Web browsers act as gateways to the internet. They help us do everything online – shop, watch videos, download books, upload pictures, play games, and more. Without them, most of our internet activities wouldn't be possible. They make the internet more exciting by letting us explore all sorts of content in different ways.
In older days websites used HTTP protocol and that was stateless. This meant that the websites couldn’t store user-related information such as user’s session ID, username, etc., in the browser.
As the web started growing and started being popular, websites started storing information of user in the browser.
Ever wondered how a website recognizes you even after you've left? Sometimes you log in, and sometimes you don't have to because the website remembers you. The website remembers your preferred language, usernames, passwords etc. This magic is performed with the help of Cookies.
Cookies:
Cookies are a method for storing lightweight data on the browser in a key-value format, usable by both the client and server. After a certain time, the browser automatically deleted them.
A cookie is like a tiny package with a name, special information about you, an end date, and a size. It keeps things like your preferences and settings to make the website work better for you.
The primary method for client-side storage was using cookies, but cookies had certain limitations and drawbacks.
Here are some of the challenges and limitations that developers encountered before the Web Storage API:
- Un-encrypted Data: Cookies were transmitted over the network in plain text, which involves a security risk. Any sensitive information stored in cookies, such as user authentication tokens, could be fetched and manipulated by attackers if the communication is not encrypted by HTTPS.
- Cookie Size Limitations: The 4 KB size limit per domain for cookies imposed severe constraints on the amount of data that could be stored. This limitation was especially problematic for applications that needed to store large datasets or complex objects on the client side.
- Per-Request Overhead: As cookies were sent with every HTTP request to the server, even for static assets like images and style sheets, it created unnecessary overhead. This additional data transfer could lead to increased latency and reduced overall performance, particularly in scenarios with limited bandwidth.
- Browser Compatibility Issues: Although cookies were widely supported, there were inconsistencies in how different browsers handled them. Some browsers had more restrictive limits on the number of cookies allowed per domain or the total size of all cookies combined.
Introduction to Web Storage APIs:
In 2014, The newest version of HTML (HTML5) was released. There were new tools and a different way to save information in the browser. This is where the Web Storage API comes into play!
Web Storage was introduced with HTML5. It's like a cool tool for web browsers, making websites and apps remember things on your device so that when you return, everything is still there.
Think of it like a magic box where websites can keep information in the form of key/value pairs. Sometimes people also call it DOM storage.
Advantages of Web Storage:
- Bandwidth Reduction: Web Storage data is stored only on your device, not sent/back and forth with every request, this results into saving the internet bandwidth and usage.
- Size Limit: You can store more significant amounts of data, up to 5MB per website.
- Data Type Support: While it mainly supports strings, you can use tricks like serializing the data by using JSON.stringify() to store different types of data into string format.
- Expiration Date: You can keep data permanently. Data stays until you decide to clear your browser. (Depending upon the type of Web Storage method)
Types of Web Storage API:
The Web Storage API provides mechanisms for storing data in browsers, such as:
- Local Storage
- Session Storage
- Local Storage:
Local Storage is like a small storage space in your web browser where websites can keep little pieces of information. This storage is useful for saving things like user preferences or data that a website wants to remember, even if you close the browser and come back later.
You can think of it as a box where you can store things with labels. To store data, use setItem(key, value), and to retrieve it, use getItem(key). However, it's important to note that everything in this box is in the form of text, so if you want to store something more complex like a list or an object, you have to convert it to text first(by using JSON.stringify()) and then convert it back (by using JSON.parse()) when you take it out.
Keep in mind that since this box is in your browser, any JavaScript code running on the same website can peek inside it. So, it’s not recommended to store sensitive information such as passwords. Despite its simplicity, local Storage is handy for making web pages more interactive and user-friendly.
How to Use Local Storage:
- Setting Data into Local Storage:
In local Storage, the setItem() method is used to store data. It requires two parameters – a key and a value. In the language of JavaScript, it looks something like this:

In this operation, the key "myKey" acts as a unique identifier, and "myValue" represents the actual data associated with that key.
- Getting Data from Local Storage:
When it's time to retrieve stored data, we can use the getItem() method. It takes only one parameter – the key whose associated value we're interested in.

This method fetches the value linked to "myKey" and assigns it to the "myThing" variable.
- Removing Data Entry From Local Storage:
In scenarios where specific data needs to be removed from Local Storage, the removeItem() method comes into play.

- Clearing All The Data From Local Storage:
For a clean slate and wiping out all stored data, the clear() method is utilized:

This action eradicates all key-value pairs stored in Local Storage, essentially resetting it to an empty state. It provides a comprehensive approach for initializing data.
Advantages:
- Persistence Across Sessions:
- Benefit: Local Storage retains data even when the user closes the browser or navigates away from the page.
- Use Case: Ideal for storing long-term preferences, user settings, or any data that needs to persist across multiple visits.
- Sufficient Storage Capacity:
- Benefit: Provides a larger storage capacity (typically around 5-10 MB per domain) compared to traditional cookies.
- Use Case: Suitable for applications requiring substantial data storage on the client side.
- Efficient Data Handling:
- Benefit: Data stored in Local Storage doesn't need to be sent to the server with every HTTP request, reducing bandwidth usage.
- Use Case: Enhances website performance by minimizing unnecessary data transmission.
- Simplicity:
- Benefit: Local Storage operations involve a straightforward API (Application Programming Interface) with methods like setItem() and getItem().
- Use Case: Easy to implement and suitable for developers of various skill levels.
Disadvantages:
- Security Concerns:
- Problem: Since data is stored locally, there are potential security risks, especially if sensitive information is stored without proper encryption.
- Consideration: Developers should be cautious about storing sensitive user data in Local Storage.
- String-Only Data Type:
- Problem: Local Storage primarily supports string data types, requiring additional steps (e.g., JSON.stringify) for complex data structures.
- Consideration: Developers must handle data serialization and deserialization for non-string data types.
- Session Storage:
Session Storage is a type of web storage in browsers that provides a temporary and isolated storage space for web applications. It allows JavaScript to store data during a user's session on a specific website, with the data automatically cleared when the session ends, typically when the user closes the browser tab or window.
This storage mechanism is designed to hold small amounts of data in the form of key-value pairs, like Local Storage. The key distinction lies in its transient nature; Session Storage is scoped to a single page session, offering a way to maintain state and preferences temporarily. Session Storage is like a temporary memory space in your web browser.
For Example, imagine you're shopping online. Websites might use Session Storage to monitor the contents of your virtual shopping cart. As long as you're on the shopping site, the cart keeps filling up. But once you close the browser or finish your shopping and leave the site, the browser tosses out that temporary shopping list.
How to Use Session Storage:
- Setting Data into Session Storage:
Session Storage provides a method called setItem() that allows you to store data for the duration of a user's session on a particular website. This method takes two parameters: a key, which acts as an identifier, and a value, which represents the data you want to associate with that key.

- Getting Data from Session Storage:
When it's time to retrieve stored data, from Session Storage, you can use the getItem() method. This method accepts key as an input parameter and returns the value associated with the key.

This function searches the value stored under “key” and map it to “storedValue” variable
- Removing Data Entry from Session Storage:
In scenarios where specific data needs to be removed from Session Storage, the removeItem() method comes into play.

This command instructs Session Storage to eliminate the data associated with the key ".
It's a targeted removal operation, useful for decluttering storage.
- Clearing All the Data from Local Storage:
The clear() method allows you to wipe out all data stored in Session Storage. This is useful when you want to reset or clear all session-specific information.

This action deletes all key-value pairs stored in Session Storage.
Advantages:
- Transient Storage:
- Benefit: Session Storage is designed to be temporary and tied to a particular browser session, with data being automatically cleared when the session concludes.
- Use Case: Ideal for storing data that is only needed during the user's current visit or session.
- Simplified Cleanup:
- Benefit: No need to manually clear data; it automatically expires at the end of the session.
- Use Case: Simplifies data management for temporary information that is no longer relevant after the session concludes.
- Same-Origin Policy:
- Benefit: Adheres to the same-origin policy, meaning a web page can only access data stored by itself or by pages from the same origin.
- Use Case: It enhances security by limiting access to session data across different origins.
Disadvantages:
- Limited Persistence:
- Problem: Session Storage is limited to the duration of a single page session, making it unsuitable for storing data needed across multiple visits.
- Consideration: Developers must carefully choose when to use Session Storage based on the specific needs of the application.
- Smaller Storage Capacity:
- Problem: Offers a smaller storage capacity compared to Local Storage, limiting the amount of data that can be stored.
- Consideration: May not be suitable for applications requiring extensive client-side data storage.
- Same-Origin Limitation:
- Problem: The same-origin policy can be a limitation if data needs to be shared between pages from different origins.
- Consideration: Developers should be aware of the restrictions when designing applications with cross-origin data requirements
Sample Implementation of Local and Session Storage into Lightning Web Components:
Markup:
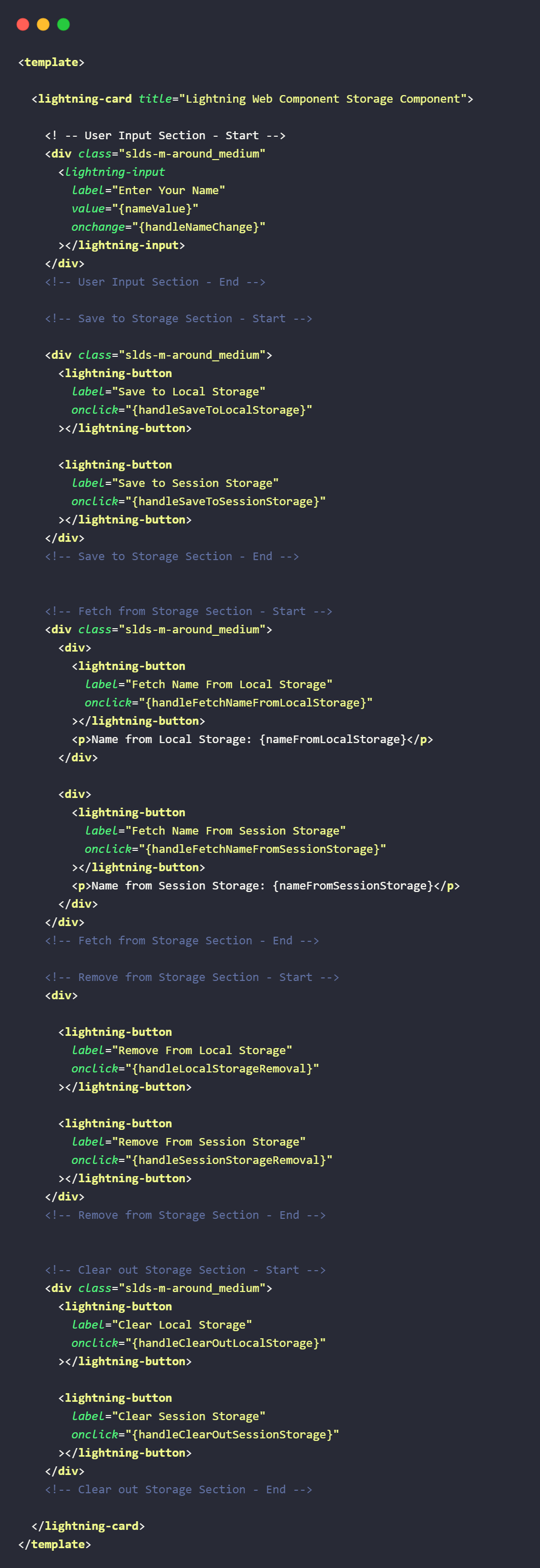
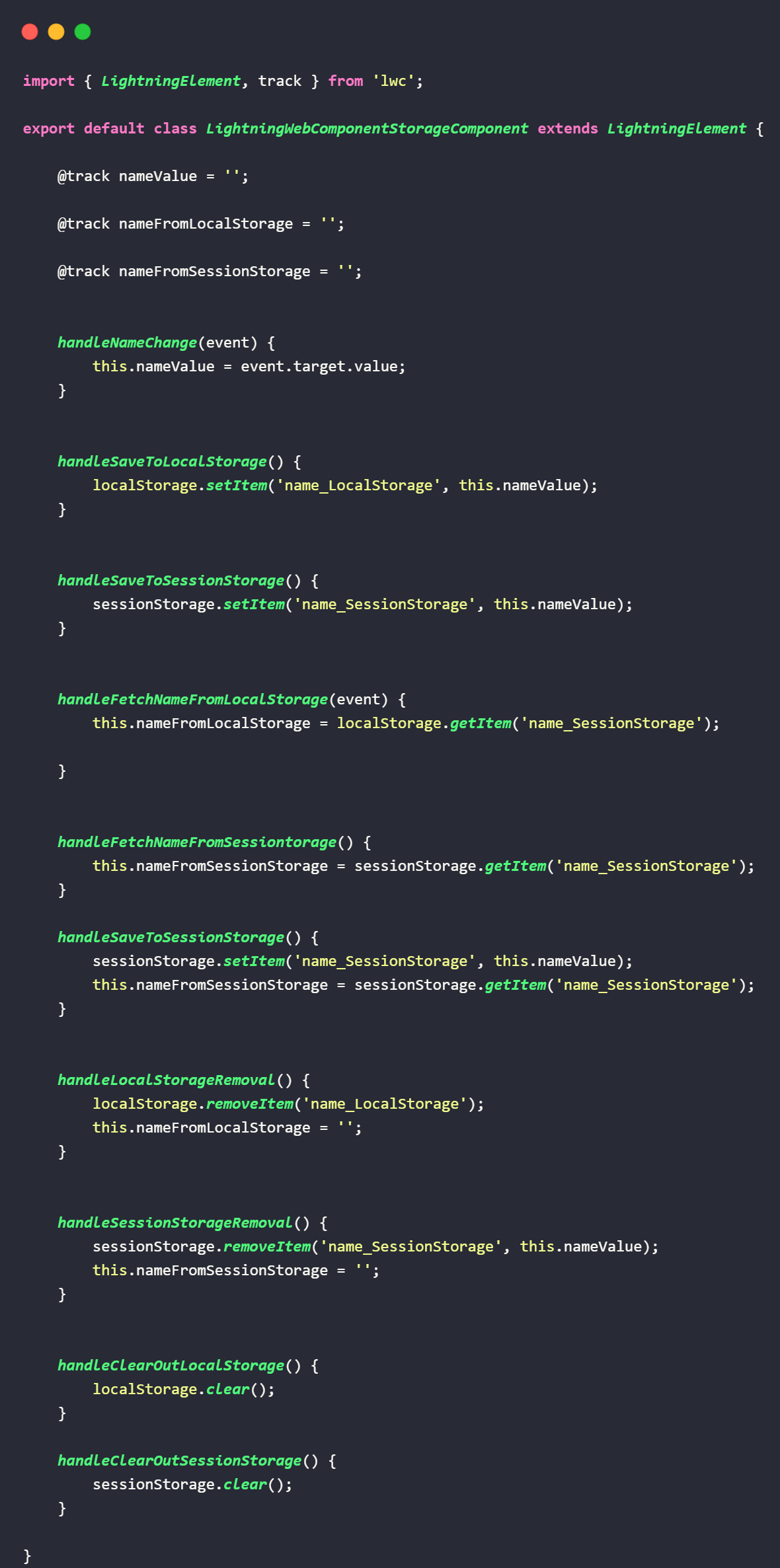
Conclusion:
In summary, Local Storage and Session Storage each have their strengths and weaknesses, and the choice between them depends on the specific requirements of the application. Local Storage is well-suited for persistent data storage, while Session Storage is designed for temporary data needs within a single session.
Developers should carefully consider factors such as security, data persistence, and storage capacity when deciding which web storage solution to employ.
Blueflame Labs offers expert web development services that leverage the power of Local and Session Storage to create exceptional user experiences. By partnering with Blueflame Labs, you can harness the full potential of Local and Session Storage to create innovative and high-performing web applications. Contact us today to learn more about our web development services.
Recent Blogs

Mastering Amortization Flow in NetSuite
Read More
Demystifying Second-Generation Managed Packages (2GP) in Salesforce
Read More
NetSuite Advanced Revenue Management (Essential)
Read More
Mastering Shipment Tracking with Zenkraft (Bringg)
Read More
Integrating Form Titan with Salesforce
Read More
Leveraging Rootstock ERP for your Purchase Orders & Authorizations
Read More
A Guide to NetSuite Bank Reconciliation
Read More
Master Google Tag Manager & Google Analytics 4
Read More