High-Performance APIs with .NET 8
Introduction
APIs are essential components of modern online applications, facilitating communication between different software systems. The release of .NET 8 brings new features and enhancements designed to boost API performance and scalability. In today's fast-paced web development landscape, the efficiency of APIs significantly impacts user experience and satisfaction. Microsoft has introduced several changes in .NET 8 to enhance API speed. In this blog, we will explore how to build high-performance APIs with .NET 8, covering various techniques and best practices.
Why Performance Matters
API performance is essential for several reasons:
- User Experience: Fast APIs ensure a smoother and more responsive experience for users.
- Scalability: High-performance APIs can manage a higher volume of requests per second, enhancing scalability.
- Cost Efficiency: Efficient APIs decrease server load, leading to reduced infrastructure costs.
New Features in .NET 8 for API Performance
HTTP/3 Support
.NET 8 comes with built-in support for HTTP/3, the latest iteration of the HTTP protocol. HTTP/3 has some advantages, such as:
- Enhanced Reliability: QUIC incorporates features like built-in error correction, boosting reliability on unstable networks.
Performance Improvements in the Core Framework
.NET 8 introduces several under-the-hood performance improvements, including:
- Faster JSON Serialization/Deserialization: Enhanced performance in processing JSON, a widely used data format in APIs.
- Optimized Memory Usage: Improved memory management to reduce the overhead of API requests.
Minimal APIs Enhancements
- Efficiency: Write less, accomplish more—a significant advantage for today's developers.
- Performance: Minimal APIs are efficient, fast, and ideal for high-performance scenarios.
- Ease of Use: They are intuitive and simple to comprehend
- Flexibility: Suitable for a range of applications, from microservices to large-scale systems.
We'll explore some examples of Minimal APIs in .NET 8 using OpenAPI -
To use OpenAPI documentation features, you'll need the Microsoft.AspNetCore.OpenApi package. Install the NuGet Package using Package Manager Console using following command:

This package is essential for utilizing the WithOpenApi extension methods demonstrated in the examples below.
Example 1: Hello World!
This API returns a "Hello World!" response. Your API greets the world at http://localhost:<port>/. Note that the port number may vary based on your settings.
Below is the code for your reference:-
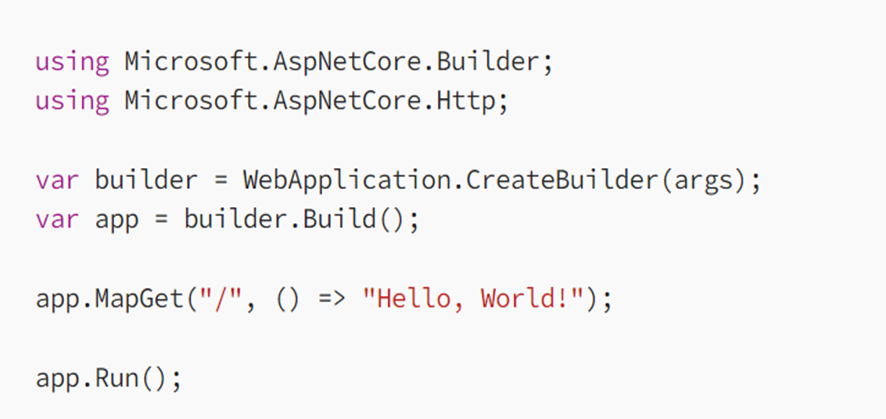
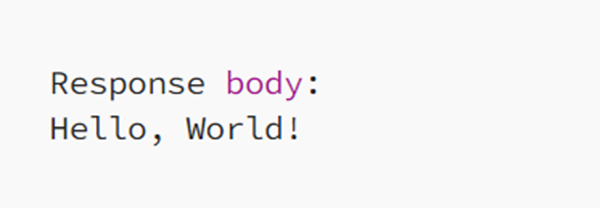
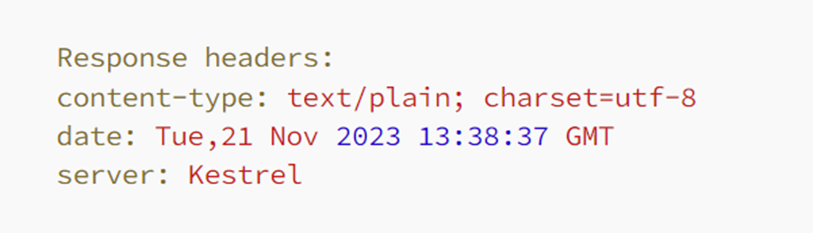
Before diving into the routes, let's review the setup. We have created an IBookService interface and its implementation, BookService, to simulate a book database. This method demonstrates how to encapsulate data access logic within a service layer, supporting separation of concerns and improving code maintainability.
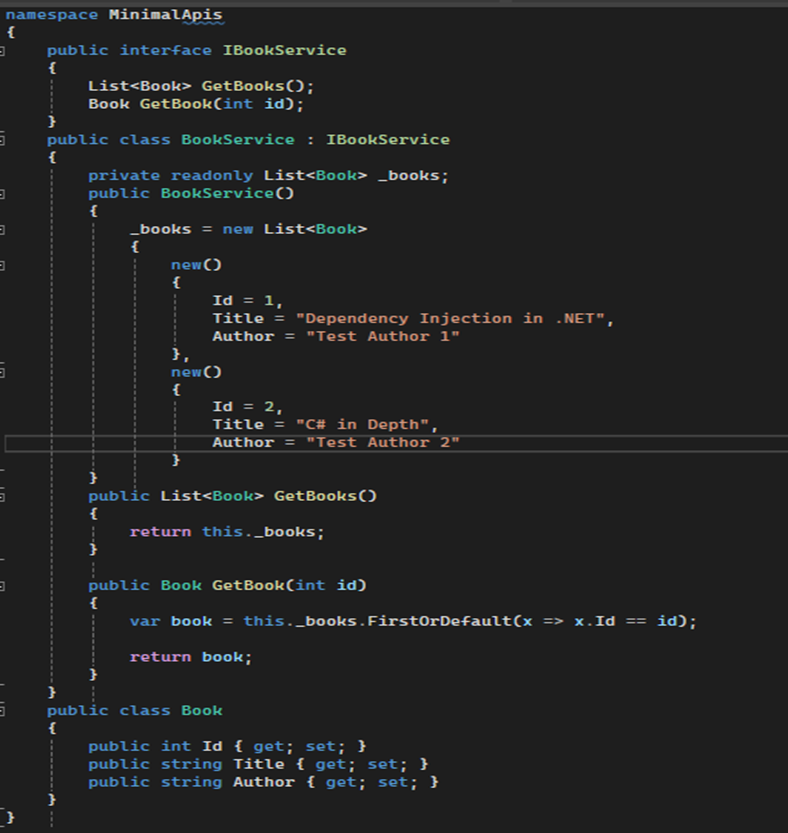
The IBookService interface serves as our blueprint, outlining the functionalities our service must provide—listing all books and fetching a specific book by ID. BookService is the concrete implementation, detailing how these functionalities operate.
Within BookService, we have a private list _books that functions as our mock database. It's pre-populated with a few books for simulation purposes, allowing us to focus on the API functionality without worrying about actual database connections.
The GetBooks method retrieves all books, and GetBook uses LINQ to find a book by its ID. This is a simple yet effective way to manage our data.

Finally, we add our BookService to the Minimal API pipeline by registering it with the dependency injection system, making it accessible throughout our application as needed.
Example 2: Fetching All Books
This example shows the list of all books in the library.
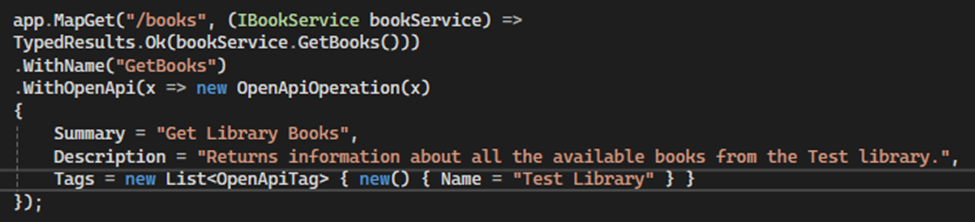
- Endpoint Definition: app.MapGet("/books", ...): This line sets up a GET endpoint at the URL /books.
- Dependency Injection: (IBookService bookService): This automatically injects IBookService, providing access to the book service.
- Service Interaction: bookService.GetBooks() invokes the GetBooks method from BookService to obtain all books.
- Response: TypedResults.Ok(...): Encapsulates the list of books in an HTTP 200 OK response.
- You can send a GET request to https://localhost:<port>/books via Postman or a browser to receive a JSON list of all books.
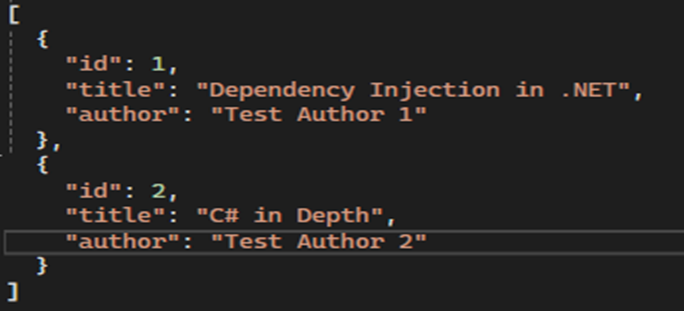
- Swagger Documentation:
- WithName("GetBooks"): Assigns a clear name to the endpoint.
- WithOpenApi(...): Adds descriptive information for the Swagger UI, improving API documentation.
Example 3: Fetches the Book by ID
This example shows how to fetch a specific book by its ID.
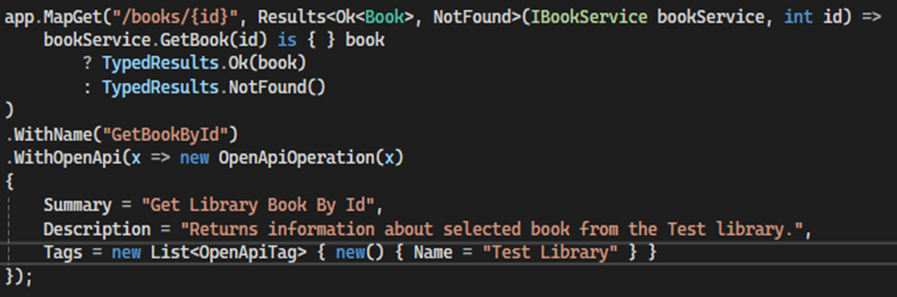
- Endpoint Definition: app.MapGet("/books/{id}", ...): Defines a GET endpoint at /books/{id}, where {id} represent the book ID.
- Route Logic: bookService.GetBook(id) is { } book: Attempts to find the book by ID. If successful, book is not null.
- Swagger Documentation: Like in Example 2, it offers valuable details for the Swagger UI.
To retrieve a book with ID 2, send a GET request to https://localhost:<port>/books/2. You will either receive the book's details in JSON format or a 404 Not Found response if the ID does not exist.
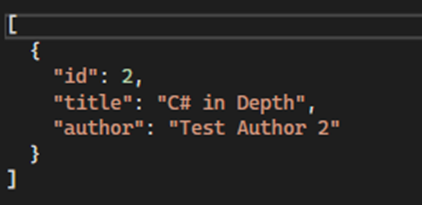
- Conditional Response:
- If found: TypedResults.Ok(book): Returns the book with an OK status.
- If the book is not found: TypedResults.NotFound() returns a 404 Not Found status.
Best Practices for Building High-Performance APIs
Use Asynchronous Programming
Asynchronous programming is vital for developing high-performance APIs. By using the async and await keywords, you can ensure that your API can handle more concurrent requests without blocking threads.
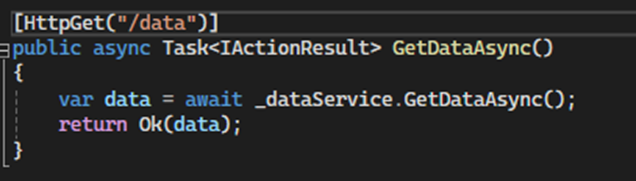
Caching Strategies
Implementing effective caching strategies can significantly enhance API performance by reducing the need to repeatedly fetch data from a database or external service.
In-Memory Caching
In-memory caching stores data within the application's memory, allowing quick access to frequently requested information.
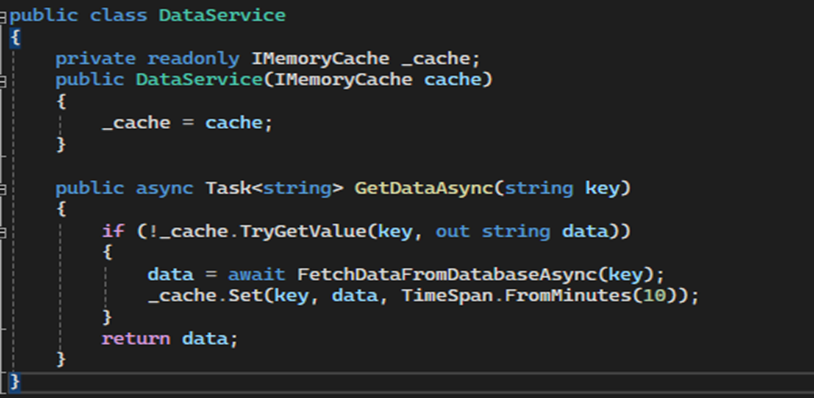
Distributed Caching
For applications running across multiple servers, solutions like Redis provide distributed caching capabilities.
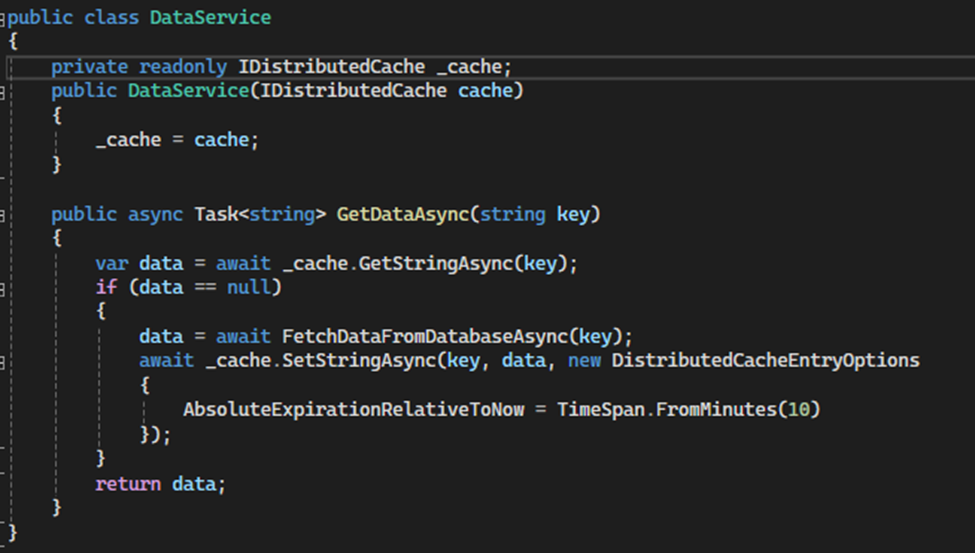
Efficient Data Access
Optimizing database access is key to API performance. Employ techniques such as:
- Connection Pooling: Minimizes the cost of establishing and terminating database connections.
- Asynchronous Database Calls: Prevents thread blocking while awaiting database operations.
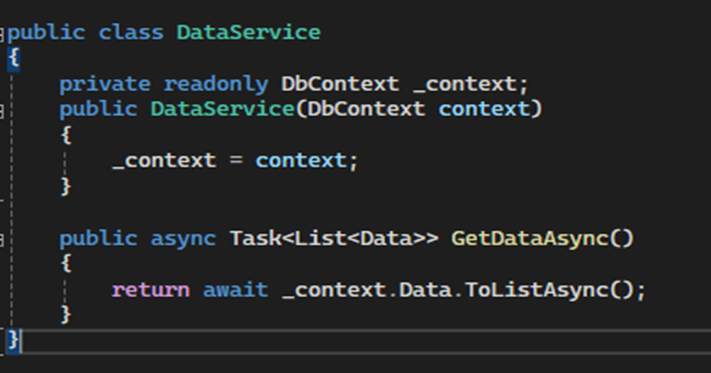
Compression
Compression reduces the size of data transmitted over the network, resulting in faster response times and decreased bandwidth usage. In .NET 8, this typically involves compressing HTTP responses to enhance the performance of web applications and APIs.
Why Compression?
- Reduced Bandwidth: Smaller payloads mean less data transfer over the network, lowering bandwidth usage.
- Faster Response Times: Compressed data travels more quickly, leading to faster client response times.
- Cost Savings: Reduced bandwidth usage can lead to cost savings, particularly for applications with high data transfer volumes.
- Improved User Experience: Faster load times enhance the overall user experience.
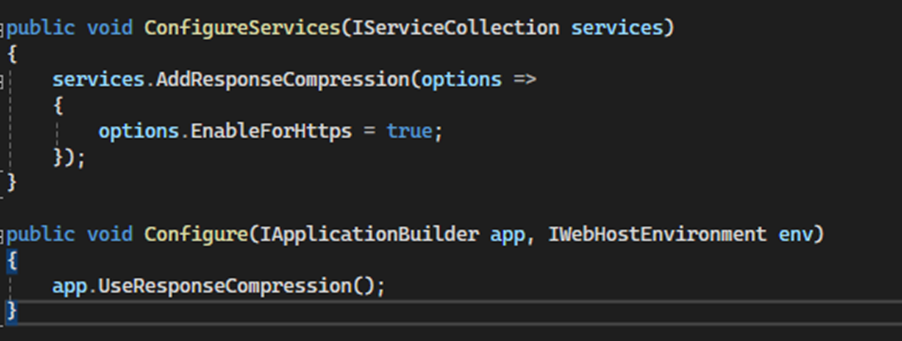
Best Practices for Compression
- Content-Type Specific Compression: Only compress content types that benefit from compression (e.g., text, JSON, XML).
- Compression Levels: Balance between compression level and CPU usage. Higher compression levels decrease the file size but result in higher CPU usage.
- Conditional Compression: Avoid compressing already compressed data formats like JPEG, MP3, or MP4.
- Monitoring and Testing: Monitor the performance impact of compression and test to ensure it improves response times without overwhelming the server.
Load Balancing
Load balancing is a technique used to distribute incoming network traffic across multiple servers. This ensures no single server becomes overwhelmed, enhances overall performance, improves scalability, and provides high availability for APIs.
Why Load Balancing?
- Scalability: Distributes the load across multiple servers, enabling the handling of more requests than a single server could manage.
- Redundancy: Ensures fault tolerance by redirecting traffic if a server fails.
- Performance: Optimizes resource use, maximizing throughput and minimizing response times.
- Availability: Ensures APIs remain accessible even under high traffic or during server maintenance.
Load Balancing Strategies
- Round Robin: Allocates requests in turn to each server on the list.
- IP Hash: Allocates requests based on a hash of the client's IP address.
- Weighted Distribution: Assigns weights to servers based on their capacity and distributes requests accordingly.
Best Practices for Load Balancing
- Health Checks: Regularly monitor the health of your servers to ensure traffic is directed only to healthy instances.
- Autoscaling: Combine load balancing with autoscaling to dynamically adjust the number of instances based on traffic load.
- Caching: Implement caching strategies to reduce the load on your API servers.
- Monitoring and Logging: Use tools like Application Insights or Prometheus to monitor performance and track issues.
Profiling and Monitoring
Profiling
To achieve optimal performance in APIs, it's crucial to understand where time and resources are spent during execution. Profiling plays a key role in gaining this understanding, and .NET 8 provides robust tools that enhance efficiency and insight.
- Visual Studio Diagnostic Tools: Visual Studio includes powerful diagnostic tools for profiling applications directly from the IDE. These tools track CPU usage, memory allocations, and garbage collection events. Developers can use them to pinpoint resource-intensive methods and optimize them for improved performance.
- .NET Profiler: The .NET Profiler offers detailed insights into the execution of .NET applications. It provides comprehensive data on method calls, execution times, and resource utilization. This tool is invaluable for identifying performance bottlenecks that might not be evident through standard debugging.
- .Net trace: In production environments where attaching a full profiler may not be feasible, dotnet-trace is invaluable. It captures diagnostic traces with minimal overhead, making it suitable for monitoring live applications. Developers can analyze these traces to uncover performance issues under real-world conditions.
- PerfView: PerfView is a powerful performance analysis tool that delves deep into CPU and memory usage patterns. It excels at identifying performance issues such as inefficient algorithms or excessive memory consumption. Its capability to handle large datasets makes it ideal for analyzing complex applications.
- BenchmarkDotNet: For optimizing specific parts of an application, BenchmarkDotNet is indispensable. It enables developers to write benchmarks that measure the performance of individual methods or code blocks. By comparing different implementations, developers can select the most efficient ones, ensuring peak performance for critical sections of their APIs.
Monitoring
While profiling aids in optimizing performance during development, monitoring is essential for maintaining consistent application performance in production. Effective monitoring enables proactive detection and resolution of performance issues, ensuring a seamless user experience.
- Application Insights: As a component of Azure Monitor, Application Insights provides extensive telemetry for .NET applications. It captures data on request rates, response times, failure rates, and more. Developers can set up alerts for anomalies, visualize trends, and analyze detailed logs to troubleshoot issues effectively.
- Prometheus and Grafana: Prometheus and Grafana provide a robust monitoring and visualization platform for those preferring open-source solutions. Prometheus collects metrics from .NET applications, while Grafana offers customizable dashboards for visualizing these metrics in real-time. This setup allows developers to effectively track and assess API performance and health.
- ELK Stack (Elasticsearch, Logstash, Kibana): Another popular open-source monitoring solution, the ELK Stack facilitates the collection, storage, and visualization of logs from .NET applications. Logstash processes logs, Elasticsearch stores them for indexing, and Kibana provides powerful search and visualization capabilities. This setup enables deep insights into application behavior and performance.
- Health Checks: .NET 8 includes built-in health check middleware, simplifying the exposure of endpoints that indicate application health status. These health checks monitor critical dependencies like databases and external services. Integrated into a comprehensive monitoring strategy, these endpoints facilitate rapid issue detection and response.
- Distributed Tracing with OpenTelemetry: In microservices architectures, distributed tracing is crucial for understanding request flows and identifying latency issues across services. OpenTelemetry integrates seamlessly with .NET, tracing requests as they traverse various services. Detailed traces provided by OpenTelemetry aid in optimizing overall system performance.
- Diagnostic Source and EventSource: .NET 8's diagnostic APIs, including DiagnosticSource and EventSource, enable developers to instrument their code for detailed monitoring and diagnostics. These APIs generate custom telemetry data that can be consumed by diverse monitoring tools, allowing for tailored monitoring solutions.

Conclusion
Building high-performance APIs with .NET 8 involves leveraging the latest features and best practices to optimize your application's performance. By incorporating HTTP/3 support, using asynchronous programming, implementing efficient caching strategies, optimizing data access, enabling compression, and employing load balancing, you can ensure your APIs are fast, reliable, and scalable. Regular profiling and monitoring are also essential to maintain optimal performance.
With these techniques, you can take full advantage of the enhancements in .NET 8 to deliver high-performance APIs that meet the demands of modern applications.
Blueflame Labs: Your Partner in API Excellence
Blueflame Labs specializes in crafting high-performance APIs using the latest .NET 8 technologies. Our team of experts can help you:
- Optimize API Performance: Identify and eliminate bottlenecks to maximize speed and efficiency.
- Leverage .NET 8 Features: Take full advantage of new features like HTTP/3, JSON improvements, and Minimal APIs.
- Implement Best Practices: Follow industry-proven strategies for building robust and scalable APIs.
- Provide Expert Consulting: Offer guidance and support throughout your API development journey.
Contact Blueflame Labs today to discuss your API performance needs and learn how we can help you achieve your goals.
Recent Blogs

Mastering Amortization Flow in NetSuite
Read More
Demystifying Second-Generation Managed Packages (2GP) in Salesforce
Read More
NetSuite Advanced Revenue Management (Essential)
Read More
Mastering Shipment Tracking with Zenkraft (Bringg)
Read More
Integrating Form Titan with Salesforce
Read More
Leveraging Rootstock ERP for your Purchase Orders & Authorizations
Read More
A Guide to NetSuite Bank Reconciliation
Read More
Master Google Tag Manager & Google Analytics 4
Read More