Secure your ASP.NET Web Application & API Part 2
Implementation of Role-Based Authorization
Implementing role-based authorization in .NET involves several steps. Here's a simplified guide:
Step 1: Define Roles
Identify the roles that users can have in your system, such as "Admin", "User", "Manager", etc. Determine the permissions associated with each role.
Step 2: Assign Roles to Users
Associate roles with users in your system. You can do this either when you sign up or through an admin panel.
Step 3: Configure Authorization Policies
Define authorization policies based on roles using the Microsoft.AspNetCore.Authorization namespace.
Step 4: Create Role Requirements
Implement custom authorization requirements that check if the user has a specific role.
Step 5: Implement Role-Based Authorization Handlers
Develop authorization handlers that evaluate the user's roles against the required roles defined in the policies.
Step 6: Apply Authorization Policies to Controllers or Actions
Apply the authorization policies to controllers or actions in your application using attributes like [Authorize(Roles = "Admin")].
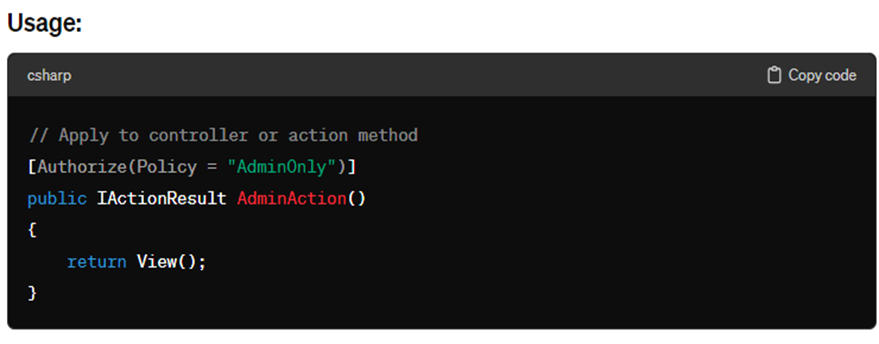
Harnessing Claims-Based Authorization
Conceptual Framework
Claims-based authorization revolves around the concept of granting access based on user attributes or claims. Unlike role-based authorization, which relies on predefined roles, claims-based authorization offers a more flexible and context-aware approach to access control.
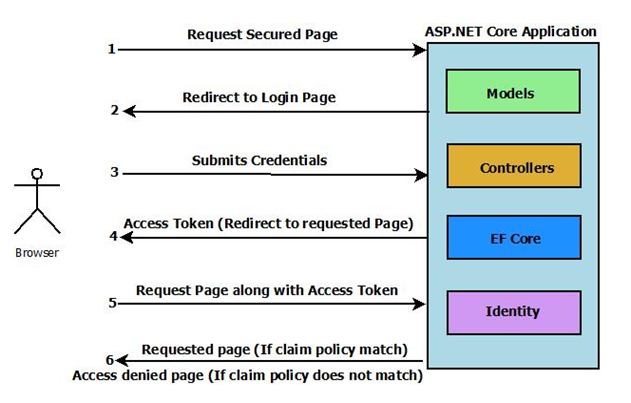
Core Components
- Claims: Assertions or attributes associated with a user, such as role memberships, department affiliations, or custom metadata.
- Policy Evaluation: Dynamically evaluating access policies based on the presence or absence of specific claims.
Implementation Strategies
- Attribute-Based Access Control (ABAC): Defining access policies based on user attributes and contextual factors.
- Policy Enforcement Points (PEPs): Enforcing access policies at various entry points within the API infrastructure, such as API gateways or middleware.
Benefits
- Adaptability: Claims-based authorization adapts dynamically to changing user attributes or contextual factors, ensuring flexibility and responsiveness.
- Fine-Grained Control: Organizations can enforce access policies based on a wide range of user attributes, allowing for precise control over API access.
- Compliance: Claims-based authorization facilitates compliance with regulatory requirements by enabling organizations to tailor access policies to specific use cases or data sensitivity levels.
Implementation
Implementing claims-based authorization in .NET involves configuring and validating claims associated with users. Here's a simplified guide:
Step 1: Define Claims
Identify the claims relevant to your application. Claims represent attributes about the user, such as roles, permissions, or custom properties.
Step 2: Assign Claims to Users
Associate claims with users during authentication or user registration. Claims can be assigned based on user attributes, roles, or other contextual information.
Step 3: Configure Authorization Policies
Define authorization policies based on claims using the Microsoft.AspNetCore.Authorization namespace.
Step 4: Create Claim Requirements
Implement custom authorization requirements that check if the user possesses specific claims.
Step 5: Implement Claims-Based Authorization Handlers
Develop authorization handlers that evaluate the user's claims against the required claims defined in the policies.
Step 6: Apply Authorization Policies to Controllers or Actions
Apply the authorization policies to controllers or actions in your application using attributes like [Authorize(Policy = "ClaimPolicy")]
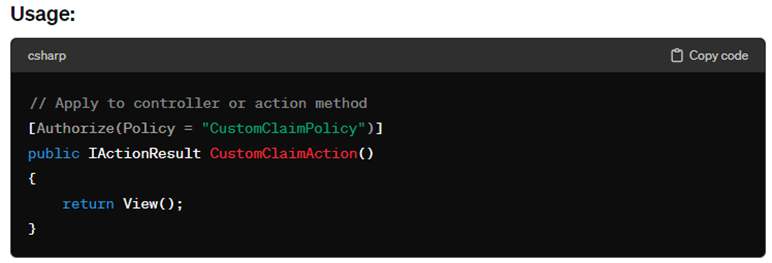
Ensuring Data Integrity with Input Validation
Input validation is a critical aspect of ensuring the integrity and security of data in software applications. It involves verifying that user input meets certain criteria or constraints before processing it further. In .NET, data annotation attributes such as [Required], [StringLength], and [Range] are commonly used to enforce validation.
- [Required]: This attribute specifies that a property must have a non-null value.
- [StringLength]: It specifies the maximum and minimum length constraints for strings.
- [Range]: This attribute constrains numeric properties to a specified range of values.
These attributes are typically applied to model properties within ASP.NET MVC or ASP.NET Core applications. They help developers define validation rules declaratively, which simplifies maintenance and promotes code readability.
Leveraging Data Annotation Attributes
In the .NET ecosystem, input validation is often implemented using data annotation attributes. These attributes provide a declarative way to define validation rules directly within your model classes, simplifying the validation process and promoting code readability. Let's explore some commonly used data annotation attributes:
- [Required]: The [Required] attribute specifies that a property must have a non-null value. It's particularly useful for ensuring that mandatory fields in your application's forms are filled out by users.
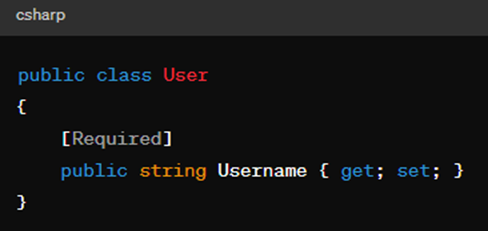
2. [StringLength]: The [StringLength] attribute constrains the length of a string property within a specified range. This helps prevent issues such as buffer overflows and database column overflows.
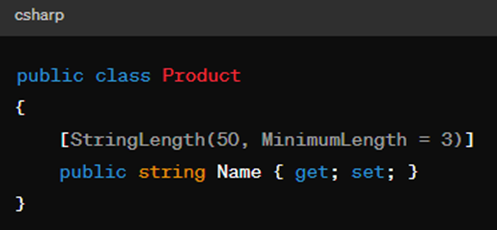
3. [Range]: The [Range] attribute validates that a numeric property falls within a specified range of values.
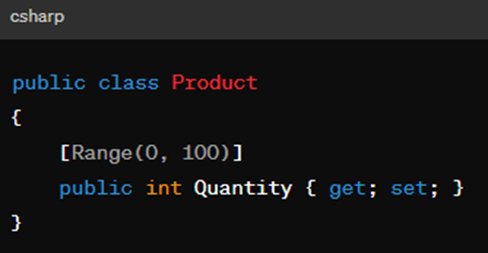
Shielding Against XSS Attacks with HTML Sanitization
Additionally, HTML sanitization is crucial for preventing cross-site scripting (XSS) attacks in web applications. The HtmlSanitizer library in .NET offers a convenient way to sanitize HTML input by removing potentially malicious content such as JavaScript, inline styles, or other dangerous elements.
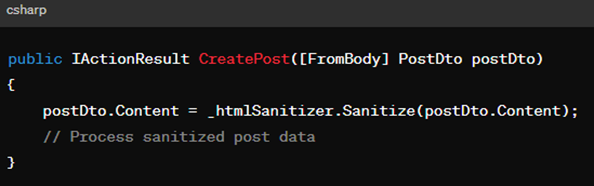
By integrating HtmlSanitizer into your application, you can ensure that user-generated HTML content is safe to display without compromising security. This is particularly important when dealing with user-generated content in forums, comments, or any other platform where HTML input is accepted and rendered. Overall, leveraging data annotation attributes for input validation and incorporating HtmlSanitizer for HTML sanitization are effective strategies for enhancing the security and robustness of .NET applications. rules on model properties
Implementing CORS
Cross-Origin Resource Sharing (CORS) is a crucial aspect of modern web development, especially when dealing with APIs that need to be accessed by clients from different origins. In .NET, implementing CORS involves configuring the application to include specific HTTP headers that allow or restrict cross-origin requests. Let's walk through the steps to implement CORS in a .NET application.
Step 1: Install the Microsoft.AspNetCore.Cors package
If you're using ASP.NET Core, you'll need to install the Microsoft.AspNetCore.Cors package. You can do this via the NuGet Package Manager or by using the .NET CLI:

Step 2: Configure CORS in Startup.cs
In your Startup.cs file, you'll need to configure CORS services and policies in the ConfigureServices method and use CORS middleware in the Configure method.
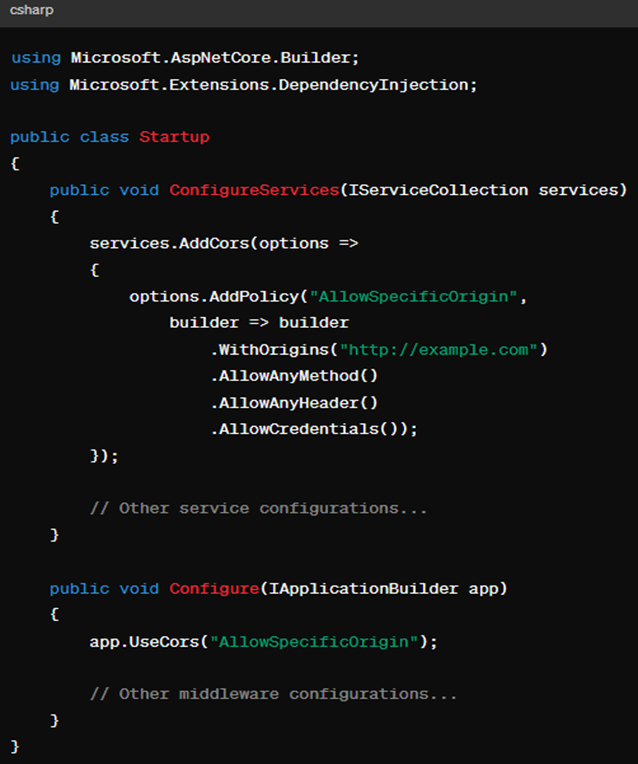
In this example, we've configured a CORS policy named "AllowSpecificOrigin" that allows requests from http://example.com with any HTTP method and headers. The AllowCredentials method is used to allow credentials (cookies, HTTP authentication) to be included in cross-origin requests.
Step 3: Apply CORS Policy to Controllers or Actions
You can apply the CORS policy globally to all controllers or selectively to specific controllers or actions by using the [EnableCors] attribute.
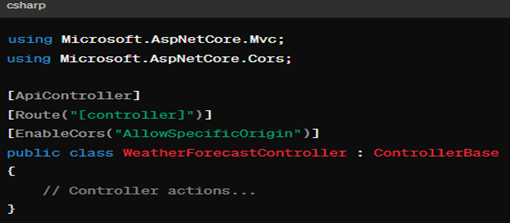
Step 4: Testing
Once CORS is configured, you can test it by sending requests from different origins to your application endpoints. Ensure that the appropriate CORS headers (Access-Control-Allow-Origin, Access-Control-Allow-Methods, Access-Control-Allow-Headers, etc.) are present in the responses.
Using Secure Headers
Securing web applications involves not only protecting data but also safeguarding against various types of attacks, including Cross-Site Scripting (XSS) and Clickjacking. Secure headers such as X-XSS-Protection and X-Frame-Options play a vital role in enhancing the security posture of your application by mitigating these threats. In .NET, you can easily add these headers to your application's responses to bolster its security. Let's walk through the process.
Step 1: Understand the Headers
- X-XSS-Protection
This header is a built-in mechanism in most modern web browsers that helps mitigate XSS attacks. It enables the browser's XSS filter, which detects and prevents reflected XSS attacks by sanitizing the page before rendering it.
- X-Frame-Options
This header helps prevent Clickjacking attacks by controlling whether your website can be embedded within a frame or iframe. It allows you to specify whether your content can be framed by other pages.
Step 2: Configure Secure Headers in .NET
In a .NET application, you can add these headers to all responses by using middleware. This ensures consistent application of these security measures across all endpoints.
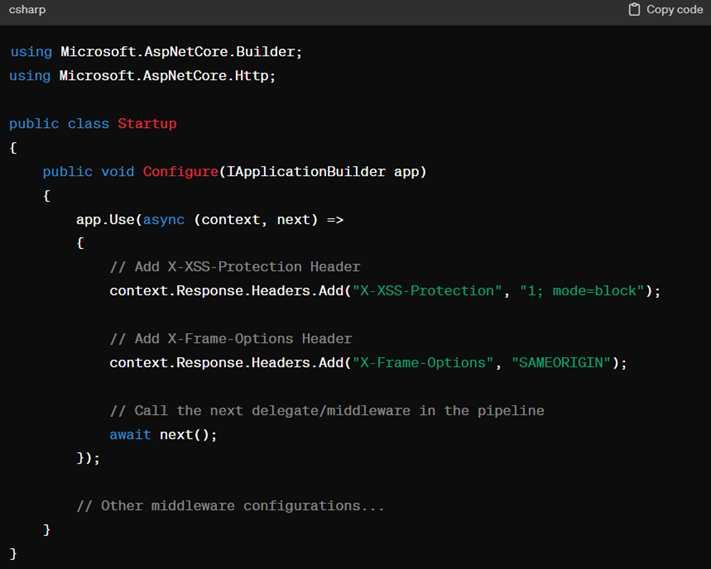
Step 3: Testing
After adding these headers, it's essential to test your application to ensure that the headers are correctly applied to all responses. You can use browser developer tools or online security scanners to verify the presence and correctness of these headers.
Implementing API Versioning and Dependency Injection in .NET Core for Backward Compatibility and Controlled Changes
When developing APIs, maintaining backward compatibility and managing changes across different versions can be challenging. API versioning helps manage these changes effectively. Additionally, implementing the Dependency Injection (DI) design pattern promotes a flexible and testable codebase. In this blog post, we will walk through the steps to implement API versioning and DI in a .NET Core application.
Adding Necessary NuGet Packages
Install the required NuGet packages for API versioning and API Explorer for Swagger integration:

Configuring API Versioning
Modify Startup.cs (or Program.cs for .NET 6 and above) to configure API versioning:
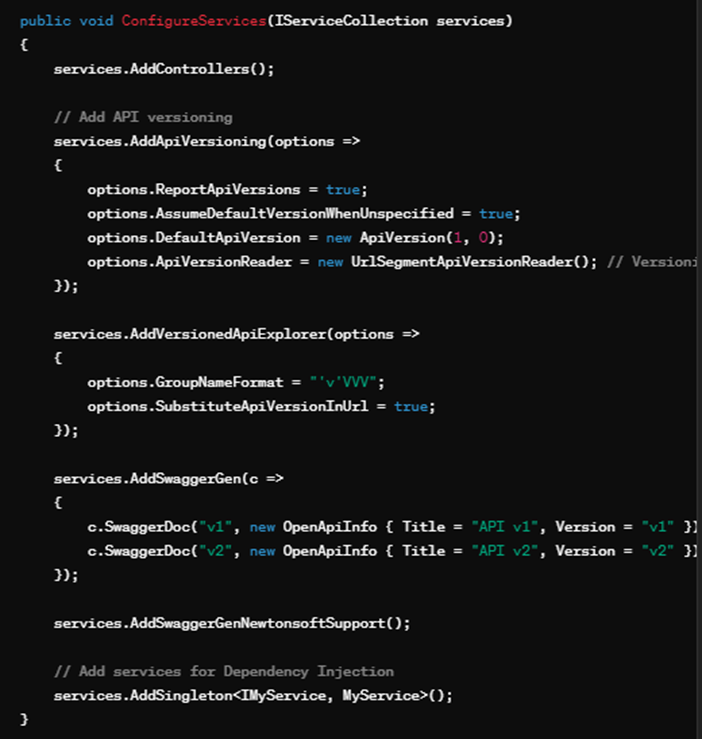
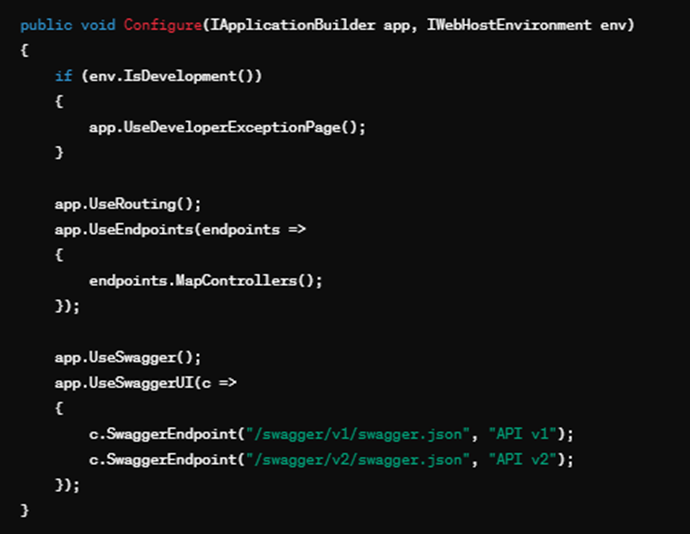
Defining API Versions
Create versioned controllers using the ApiVersion attribute:
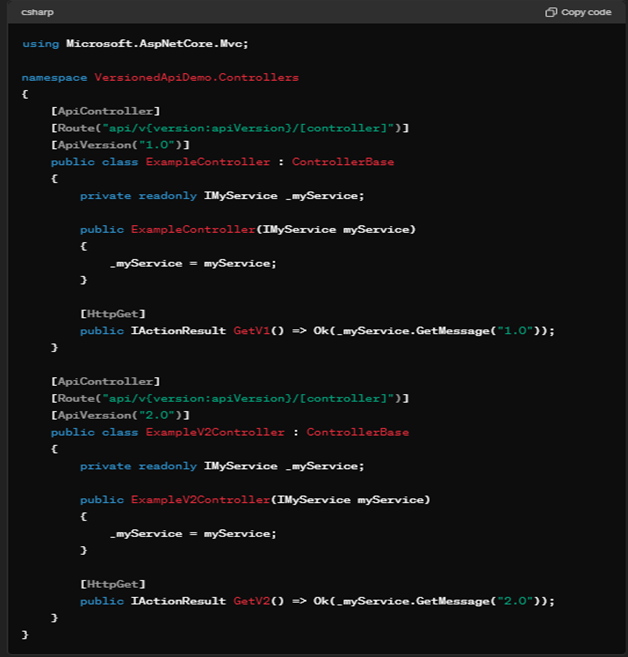
Implementing Dependency Injection
Define the service interface and its implementation:
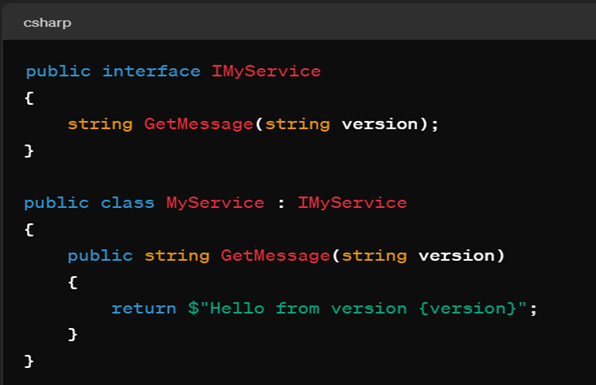
Enforcing Security Policies Based on Versions
To apply different security policies for different API versions, use custom authorization attributes:
- Create a custom authorization attribute:
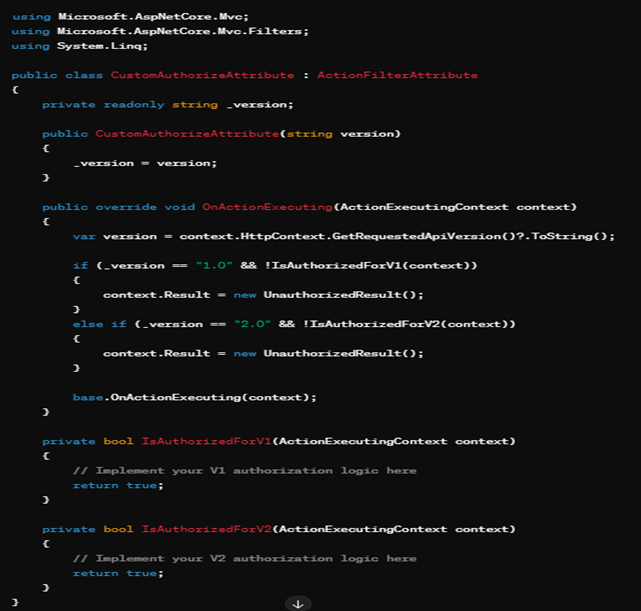
2. Apply the custom authorization attribute to your controllers:
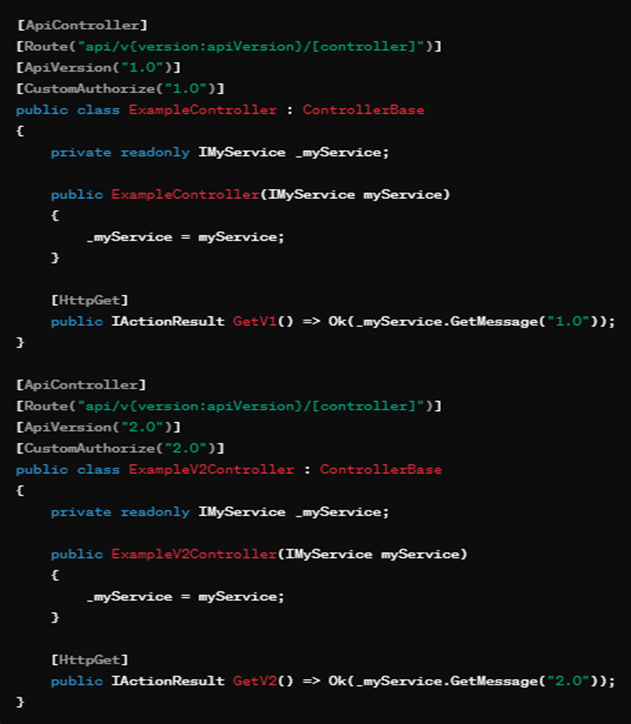
Testing Your API
Run your application and navigate to:

You should see the different responses and security behaviors for each version.
Keeping Dependencies Up to Date
Keeping your project dependencies up to date is crucial for maintaining security, performance, and accessing new features. Here's how you can manage this effectively in your .NET Core projects:
- Regularly Check for Updates: Use the .NET CLI to check for outdated packages:
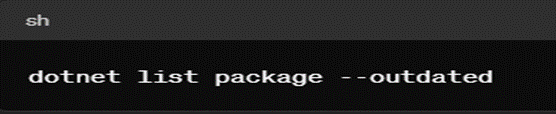
2. Update Packages: Update packages using the .NET CLI:
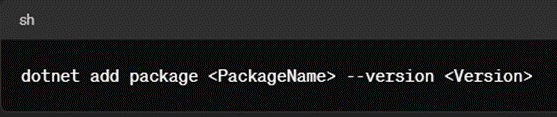
- Monitor Release Notes: Keep an eye on the release notes of the packages you use. They often contain important information about new features, bug fixes, and potential breaking changes.
- Automate Testing: Ensure you have a comprehensive suite of automated tests. This allows you to confidently update dependencies knowing that your tests will catch any issues.
- Use Semantic Versioning: Understand and respect semantic versioning used by NuGet packages. This helps in predicting the impact of updates (major, minor, patch).
Implementing Content Security Policy (CSP)
Content Security Policy (CSP) is an essential security feature designed to help protect websites from a variety of attacks, including Cross-Site Scripting (XSS) and data injection attacks. These attacks can lead to data theft, site defacement, or even the distribution of malware. By controlling which resources can be loaded on your web pages, CSP helps prevent these vulnerabilities.
In simple terms, CSP allows a web page to specify what sources of content are considered trustworthy. For instance, a page can declare which domains JavaScript, CSS, and images can be loaded from. This restriction significantly reduces the risk of malicious content being injected into the site.
CSP is enforced through the Content-Security-Policy HTTP response header, which browsers interpret to apply the specified policies.
Headers
- X-Content-Type-Options: MIME-type sniffing is a technique used by hackers to exploit missing metadata on served files. By using the "nosniff" value, we can prevent older browsers from attempting MIME-type sniffing, thereby reducing the risk of this kind of attack.

2. X-Frame-Options: Hackers may attempt to embed your website within an iframe to deceive users into clicking unintended links. To prevent this, we use the X-Frame-Options header, which informs any client that framing of your site isn't permitted. Adding this header is straightforward with middleware.
3. X-Xss-Protection: The X-XSS-Protection header instructs most modern browsers to halt page loading upon detecting a cross-site scripting attack. Adding this header can be done easily using middleware.
4. Strict-Transport-Security: ensures that all pages are served over HTTPS, enhancing security by preventing any content from being served over HTTP. While you can set the Strict-Transport-Security header using custom middleware, ASP.NET Core provides built-in middleware named HSTS (HTTP Strict Transport Security Protocol).
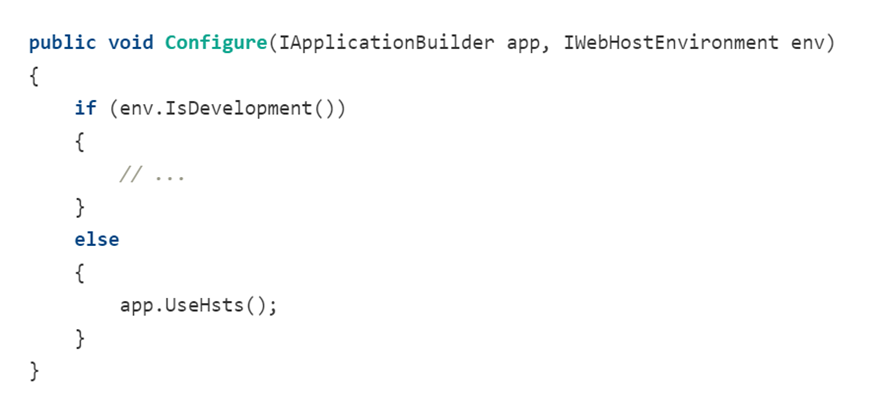
It's advisable to enable HSTS only in production environments to avoid complications during local development. You can customize the value of the Strict-Transport-Security header through options, as demonstrated in the code snippet.
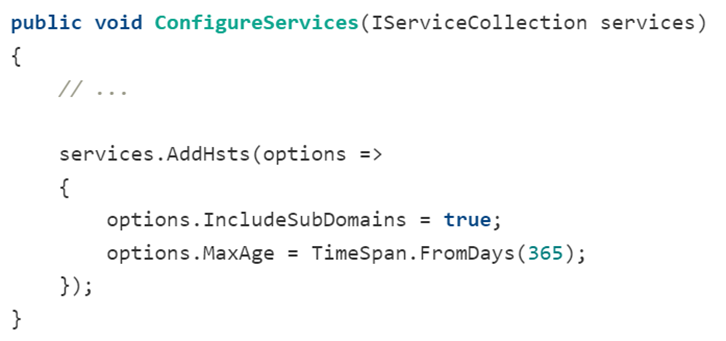
Adding CSP Response Headers in ASP.NET Core
To add CSP headers to every response in an ASP.NET Core application, you can use middleware. Middleware in ASP.NET Core is a lightweight framework for handling HTTP requests and responses. By inserting custom middleware into the request pipeline, you can add CSP headers before any other processing takes place.
Here’s a straightforward way to add CSP headers using middleware in the Startup.cs file of your ASP.NET Core application:
- Open Startup.cs: This file contains the configuration for your ASP.NET Core application.
- Add the CSP Middleware: Insert the following middleware in the Configure method. Ensure this middleware is placed before the call to UseEndpoints to make sure CSP headers are added to all responses:
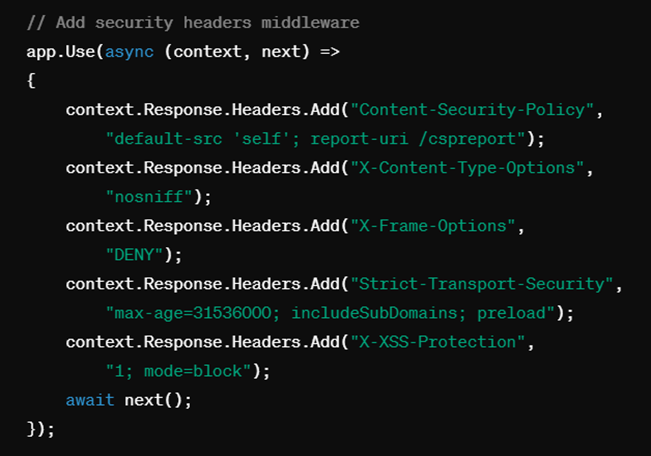
In this example, the Content-Security-Policy header is set to only allow resources to be loaded from the same origin as the page itself ('self'). Additionally, it specifies a reporting endpoint (/cspreport) where the browser can send violation reports.

When this resource is blocked, you will see an error in the browser's console, indicating that the content was not loaded due to CSP restrictions.
Testing CSP
To see CSP in action, consider trying to load an external resource that is not allowed by the policy. For example, if your CSP policy only allows resources from the same origin, attempting to load Bootstrap's CSS from a CDN would be blocked by the browser.
Protecting Your ASP.NET Core Application from Cross-Site Request Forgery (CSRF) Attacks with ValidateAntiForgeryToken
Cross-Site Request Forgery (CSRF) attacks pose a significant threat to web applications by exploiting the trust a site has in a user's browser. Attackers can trick users into unknowingly submitting malicious requests, potentially leading to unauthorized actions being performed on behalf of the victim. To mitigate this risk, ASP.NET Core provides a built-in mechanism called ValidateAntiForgeryToken. In this blog post, we'll explore how to use ValidateAntiForgeryToken to safeguard your ASP.NET Core application against CSRF attacks.
Understanding Cross-Site Request Forgery (CSRF)
CSRF attacks occur when a malicious website or attacker tricks a user's browser into sending unauthorized requests to a target web application where the user is authenticated. These requests often result in actions being executed without the user's consent, such as changing account settings, making purchases, or even performing actions with financial implications.
Introducing ValidateAntiForgeryToken
ValidateAntiForgeryToken is a security feature provided by ASP.NET Core to protect against CSRF attacks. It works by adding an anti-forgery token to forms in your application, which is validated upon form submission. If the token is missing or invalid, the request is rejected, preventing unauthorized actions from being executed.
Implementing ValidateAntiForgeryToken in ASP.NET Core
To demonstrate how ValidateAntiForgeryToken works, let's consider a scenario where we have a form in our ASP.NET Core application for updating a user's profile information.
- Add the ValidateAntiForgeryToken Attribute: Apply the ValidateAntiForgeryToken attribute to the controller action that handles the form submission:
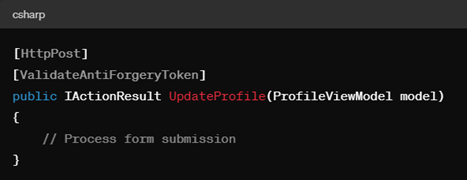
2. Include the Anti-Forgery Token in the Form: In your Razor view, include the anti-forgery token within the form using the @Html.AntiForgeryToken() helper method:
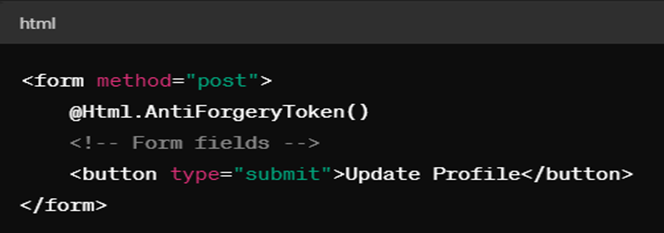
3. Configure Anti-Forgery Middleware: Ensure that the Anti-Forgery Middleware is registered in your ASP.NET Core application's Startup class:
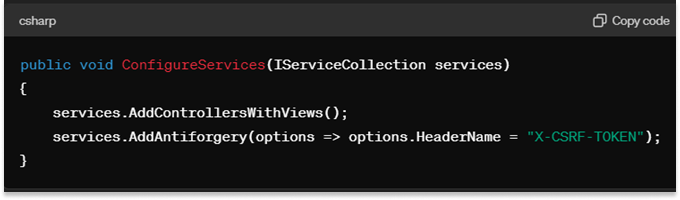
Benefits of ValidateAntiForgeryToken
- Protection Against CSRF Attacks: By requiring a valid anti-forgery token with each form submission, ValidateAntiForgeryToken effectively prevents CSRF attacks.
- Ease of Integration: ASP.NET Core provides built-in support for anti-forgery tokens, making it simple to implement CSRF protection in your applications.
- Flexibility: ValidateAntiForgeryToken can be applied to individual controller actions or globally across your application, providing flexibility in how you secure your forms.
Conclusion
To conclude, securing your ASP.NET web application and API goes beyond just basic protections. It involves implementing comprehensive authentication and authorization mechanisms, like role-based and claims-based authorization, ensuring data integrity through input validation, and preventing XSS attacks with HTML sanitization. These strategies help fortify your application's security, keeping sensitive data safe and mitigating emerging threats. As our digital landscape continues to evolve, staying proactive about API security is key to maintaining trust, integrity, and compliance. Tailor these implementations to fit your specific requirements and ensure your application's maximum security and functionality.